JavaScript SDK
Overview
The Userback JavaScript SDK provides developers with powerful tools to seamlessly integrate Userback into your application, enabling precise control and customization of the widget and feedback collection.
Prerequisites
Before you begin, ensure the following:
- Userback Workspace: A workspace is needed to collect and manage feedback.
- Access Token: Retrieve your token via the Code Icon in your workspace.
- Plan Requirements: Available on all plans; Custom Data Collection (
setData()
andcustom_data
) requires the Scale Plan or higher.
Why use the JavaScript SDK?
Powerful tools for developers to integrate Userback seamlessly into applications and do things like:
- Identify Users: Associate feedback with individual users using
identify()
oruser_data
. Personalize insights with attributes like name, email, and account details. - Control the Widget: Use methods like
show()
,hide()
, anddestroy()
to control widget visibility and behavior dynamically. - Trigger Widget Pop-ups: Open specific feedback forms or tools (e.g., screenshot, video) programmatically using
open()
. - Survey Integration: Launch and close surveys on-demand with
openSurvey()
andcloseSurvey()
. - Session Replay Management: Start, stop, and customize session replay to analyze user interactions in detail.
- Track Custom Events: Add specific events to session replays with
addCustomEvent()
for detailed behavior analysis. - Dynamic Data Handling: Capture and attach session-specific (
setData()
) or static (custom_data
) information for richer context.
(Custom Data requires the Scale Plan or higher.) - Event Handling: Leverage hooks like
on_load
orbefore_send
to trigger custom behaviors based on widget events. - Widget Customization: Modify appearance, position, triggers, and more using
widget_settings
. Adjust settings dynamically for precise control. - Environmental Adaptation: Optimize the widget for different environments (local vs. production) using options like
is_live
andnative_screenshot
.
SDK Methods
Identify Users
identify(uid: string, attributes: object)
identify(uid: string, attributes: object)
Identifies a logged-in user with unique attributes. Should be used after initializing the widget.
MyApp.on('login', () => {
Userback.identify('USR789', {
name: 'Jane Doe',
email: '[email protected]',
plan: 'Enterprise',
account_id: '789XYZ'
});
});
Note:
identify()
can be called multiple times, and it overwrites previous data.- Key names should not contain special characters.
- Values must be JSON strings, numbers, or booleans.
user_data: object
user_data: object
Use this when the JavaScript SDK hasn't been initialized. This will identify the user and provide a similar set of attributes.
Userback.user_data = {
id: 'USR789',
info: {
name: 'Jane Doe',
email: '[email protected]',
plan: 'Enterprise',
account_id: '789XYZ'
}
};
Widget Button
showLauncher()
, hideLauncher()
showLauncher()
, hideLauncher()
Show or hide the Userback widget button on the screen.
Userback.showLauncher(); // Show the widget
Userback.hideLauncher(); // Hide the widget
Widget Pop-up
openForm(type: string, destination: string)
openForm(type: string, destination: string)
Opens the widget for specified feedback type and destination. π‘ Tip: Hide the Widget Button with Widget Settings.
Feedback Types:
general
,bug
, andfeature_request
Destinations:
screenshot
,video
, andform
Userback.openForm('bug', 'screenshot'); // open the screenshot tool and then direct to the bug form
close()
close()
Closes the Userback widget popup.
Userback.close();
Widget Destroy
destroy()
destroy()
Removes the Userback widget and SDK from your application.
Userback.destroy();
Survey
openSurvey(survey_id: string)
openSurvey(survey_id: string)
Opens the specified survey. π‘ Tip: Hide the Survey with Survey Settings.
Userback.openSurvey('%%survey_id%%');
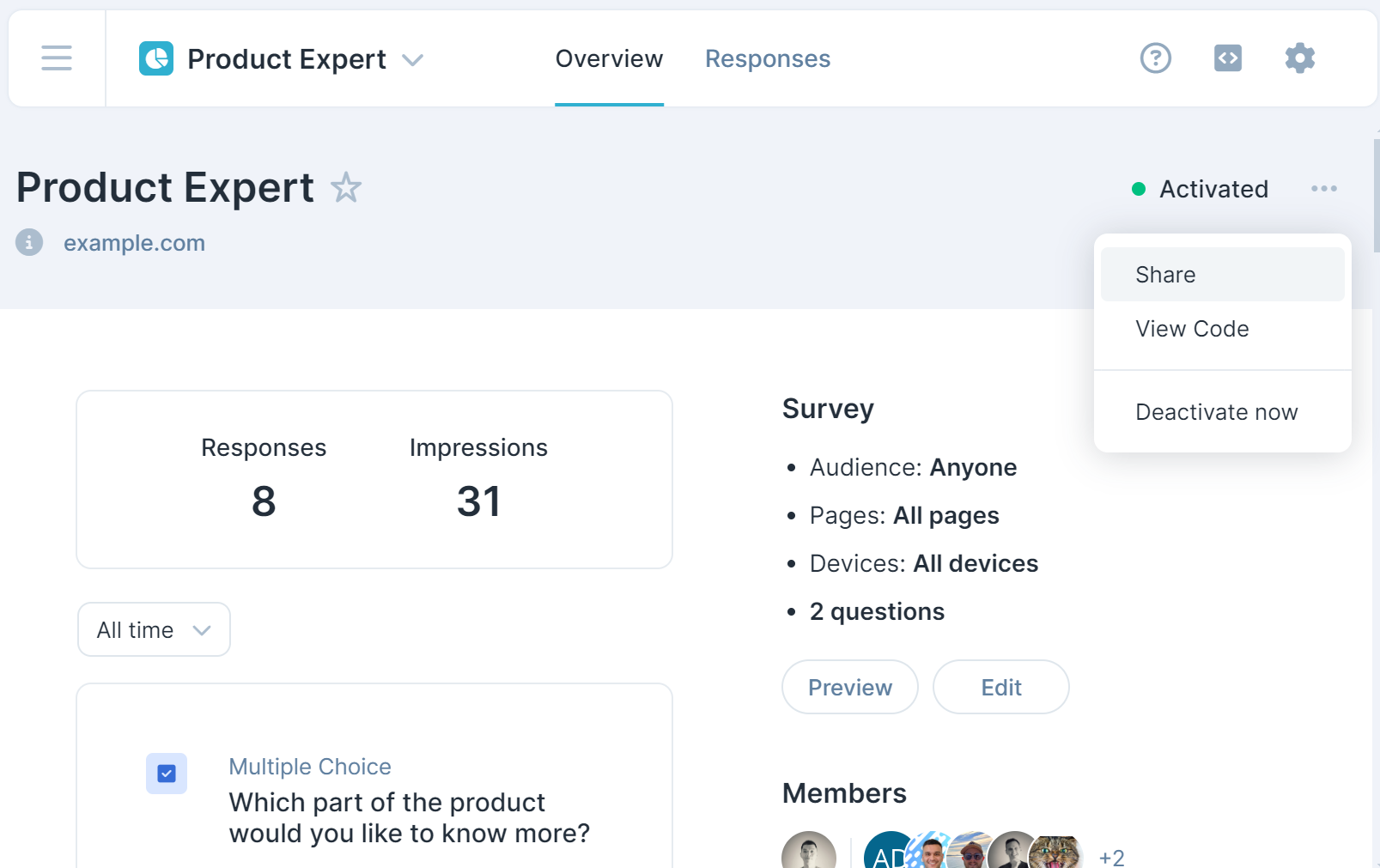
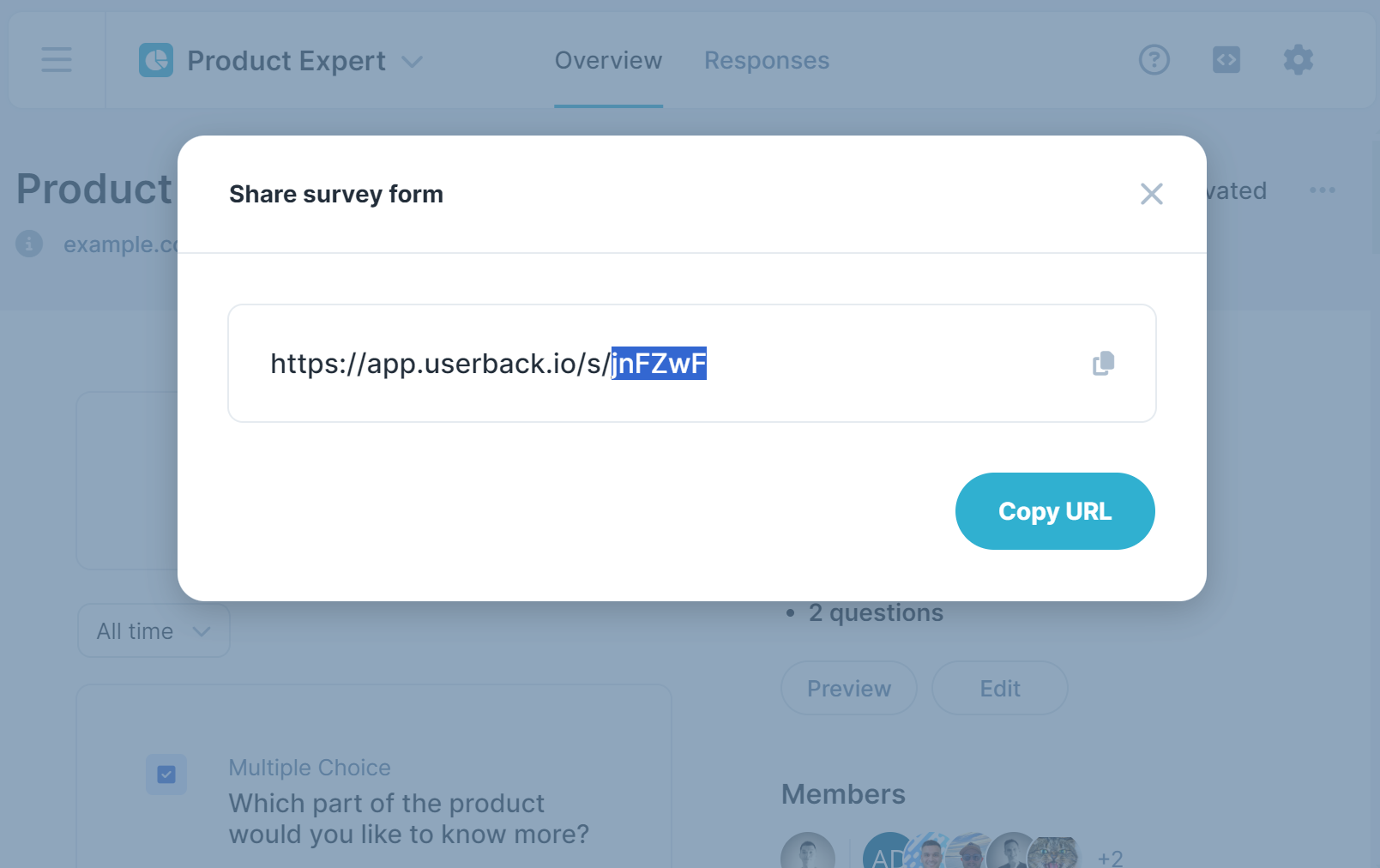
closeSurvey()
closeSurvey()
Closes the Userback survey.
Userback.closeSurvey();
Session Replay
startSessionReplay(options: object)
startSessionReplay(options: object)
When session replay is turned off for your domain, you can still start it programmatically using startSessionReplay()
.
Userback.startSessionReplay({
block_rule: '.userback-block', // optional
ignore_rule: '.userback-ignore', // optional
log_level: ['log', 'warn', 'info', 'debug', 'error'] // optional
tags: ['tag1', 'tag2'] // optional
});
stopSessionReplay()
stopSessionReplay()
Stops full session replay.
Userback.stopSessionReplay();
addCustomEvent(event_title, event_details)
addCustomEvent(event_title, event_details)
Track event in session replay.
Userback.addCustomEvent('account_upgrade', {
account_id: 123456 ,
amount: '99'
});
SDK Options
Name and Email
Automatically populate Feedback name and email fields without relying on User Identification.
email: string
email: string
Set the default email. The email field will be auto-filled in the feedback form.
Userback.email = "[email protected]";
name: string
name: string
Set the default name. The name field will be auto-filled in the feedback form.
Userback.name = "Someone";
Categories and Priority
categories: string
categories: string
Preselect the category that will be chosen in the feedback form. The value assigned must match one of the existing categories already configured in your Userback settings.
Userback.categories = "Frontend"; // Preselects the 'Frontend' category
priority: string
priority: string
Set the default priority level for feedback.
Priority Types:
low
,neutral
,high
, andurgent
Userback.priority = "urgent"; // Sets the priority to 'urgent'
Use this when the JavaScript SDK hasn't been initialized. This will identify the user and provide a similar set of attributes.
Userback.user_data = {
id: 'USR789',
info: {
name: 'Jane Doe',
email: '[email protected]',
plan: 'Enterprise',
account_id: '789XYZ'
}
};
Custom Data
setData(data: object)
setData(data: object)
Capture session-specific or dynamically changing data. Use this method after initializing the widget with init()
.
Userback.setData({
AB_test_variant: 'B',
time_on_page: '2m 15s'
});
π Important: The setData()
method is only available on the Scale Plan or higher.
Note:
setData()
can be called multiple times, overwriting previous data.- Key names should not contain special characters.
- Values must be JSON strings, numbers, or booleans.
custom_data: object
custom_data: object
- Capture static custom data when the JavaScript SDK hasn't been initialized.
Userback.custom_data = {
page_name: 'Checkout',
user_status: 'Active'
};
π Important: The custom_data
property is only available on the Scale Plan or higher.
Event Handling
on_load: function
, on_open: function
, on_close: function
, before_send: function
, after_send: function
on_load: function
, on_open: function
, on_close: function
, before_send: function
, after_send: function
Customize the widget's behavior during specific events.
Userback.on_load = () => {
console.log("Widget Loaded");
};
Build Environment
is_live: boolean
is_live: boolean
Configure the screenshot engine based on your website's hosting environment.
is_live: true
: Use this when your website is locally hosted, but assets like images and scripts are publicly accessible. Enables the live screenshot server for higher-quality captures.is_live: false
: Forces the use of the client-side screenshot engine, which may not generate pixel-perfect images. Recommended for restricted or isolated environments. Learn how to whitelist our IPs for improved quality.
Userback.is_live = true;
Native Screenshot
native_screenshot: boolean
native_screenshot: boolean
Force the browser's built-in Screen Capture API for capturing screenshots.
Userback.native_screenshot = true
Note: Useful when dealing with complex web elements like shadow DOM and iframes. This enables pixel-perfect screenshots that may not be achievable using our default screenshot engine.
Widget Settings
widget_settings: object
widget_settings: object
Dynamically configure various aspects of your widget's appearance and functionality.
Global Settings
Language (
string
): "en", "fr", "zh-CN" Language code options
Style (string
): "text", "circle"
Position (string
): "e", "w", "se", "sw"
Trigger Type (string
): "page_load", "api", "url_match"
Main Button Text (string
)
Main Button Background Colour (string
) "#000FFF"
Main Button Text Colour (string
) "#000FFF"
Device Type (string
): "desktop", "tablet", "phone"
Help Link (string
)
Help Title (string
)
Help Message (string
)
Logo (string
)
Form Settings
Rating Type (
string
): "star", "emoji", "heart", "thumb", "numbers"
Rating Help Message (string
)
Name Field (boolean
)
Name Field Mandatory (boolean
)
Email Field (boolean
)
Email Field Mandatory (boolean
)
Title Field (boolean
)
Title Field Mandatory (boolean
)
Comment Field (boolean
)
Comment Field Mandatory (boolean
)
Display Category (boolean
)
Display Feedback (boolean
)
Display Attachment (boolean
)
Display Assignee (boolean
)
Display Priority (boolean
)
Userback.widget_settings = {
language : "en",
style : "text",
position : "e",
trigger_type : "page_load",
main_button_text : "Feedback",
main_button_background_colour : "#3E3F3A",
main_button_text_colour : "#FFFFFF"
device_type : "desktop,tablet,phone",
help_link : "https://www.example.com",
help_title : "Contact Us",
help_message : "Get in touch with us",
logo : '//example.com/logo.jpg', /
form_settings: {
// General Feedback Form
general: {
rating_type : "star",
rating_help_message : "How would you like to rate your experience?",
},
// Bug Form
bug: {...},
// Feature Request Form
feature_request: {...}
}
};
π Support
Need help? Hereβs how to get assistance:
- Log in to your Userback workspace.
- Click the Help Center icon in the top-right corner.
- Select Contact us to start a chat with our support team.
Updated 9 days ago